ORM (Object-Relational Mapping) is a programming technique used to map data from relational databases into object models, enabling developers to interact with databases using an object-oriented approach. The three most popular JavaScript ORMs are Prisma, TypeORM, and Sequelize. Below is an introduction and comparison of these three ORMs.
Prisma: Modern and Lightweight
Prisma is a modern, lightweight ORM that provides out-of-the-box support for TypeScript. Its clean and intuitive syntax, combined with generated TypeScript types, ensures a highly secure development experience.
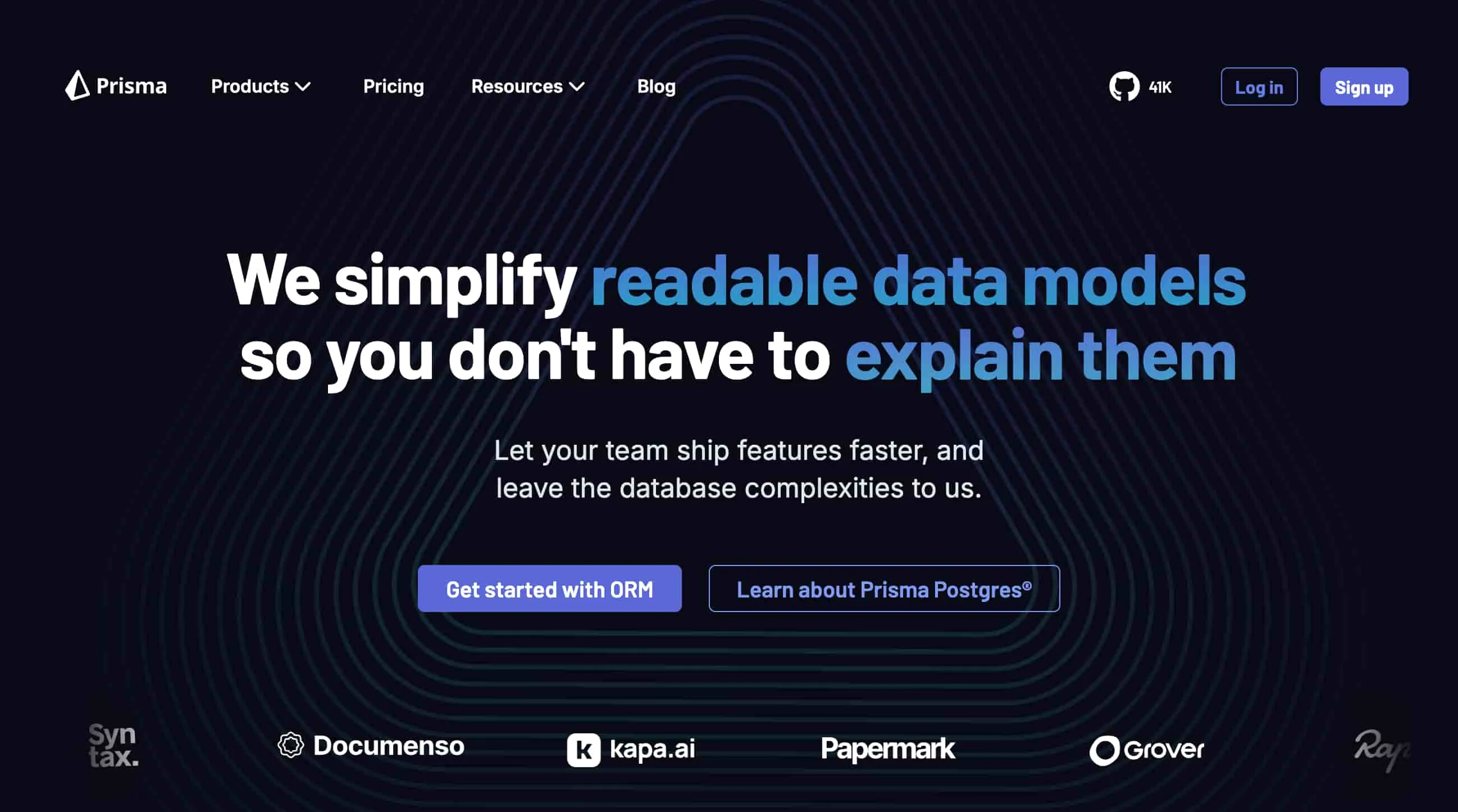
Key Features
- Emphasis on type safety.
- Declarative migrations.
- Supports multiple databases, including PostgreSQL, MySQL, SQLite, and SQL Server.
Code Example
import { PrismaClient } from "@prisma/client";const prisma = new PrismaClient();async function main() { const allUsers = await prisma.user.findMany(); console.log(allUsers);}main() .catch((e) => console.error(e)) .finally(async () => await prisma.$disconnect());
Documentation
TypeORM: Flexibility and Broad Database Support
TypeORM is another powerful ORM known for its flexibility. It supports both Active Record and Data Mapper patterns and offers compatibility with various databases.
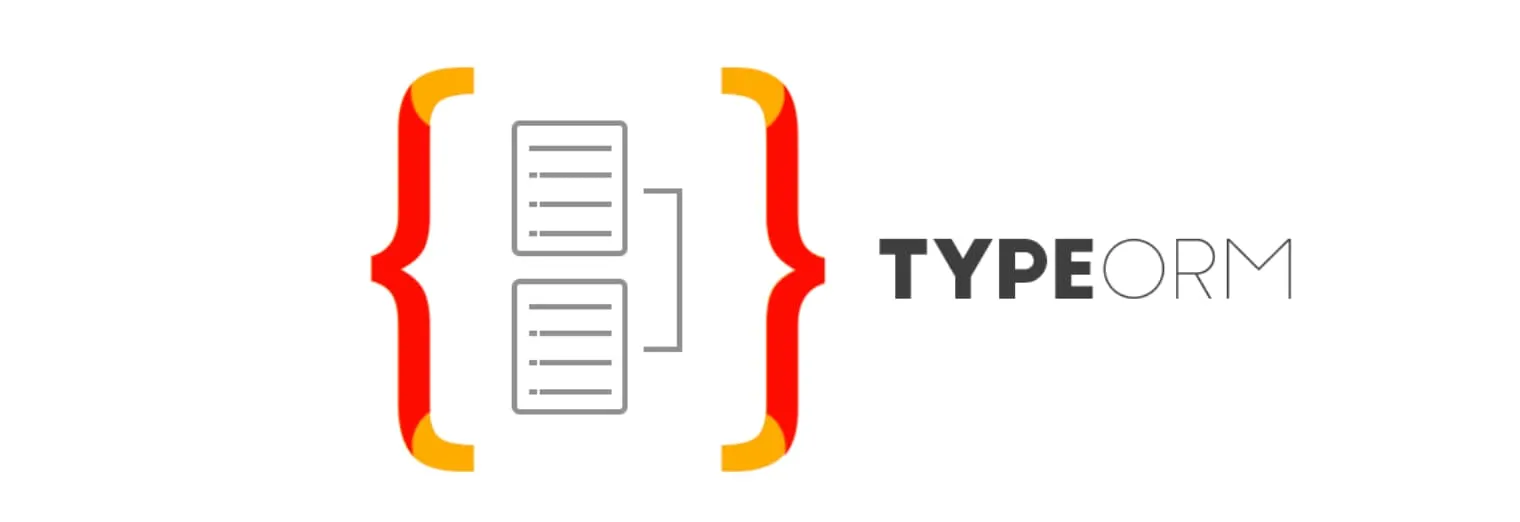
Key Features
- Support for decorators and TypeScript.
- Entity-based data modeling.
- Compatible with databases like PostgreSQL, MySQL, SQLite, and more.
Code Example
import { Entity, PrimaryGeneratedColumn, Column, createConnection } from "typeorm";@Entity()class User { @PrimaryGeneratedColumn() id: number; @Column() name: string;}async function main() { const connection = await createConnection(); const userRepository = connection.getRepository(User); const users = await userRepository.find(); console.log(users);}main();
Documentation
Sequelize: Broad Database Compatibility and Strong Community
Sequelize is a mature ORM and one of the oldest in the JavaScript ecosystem. With its Promise-based API, Sequelize excels at handling traditional database operations in Node.js.
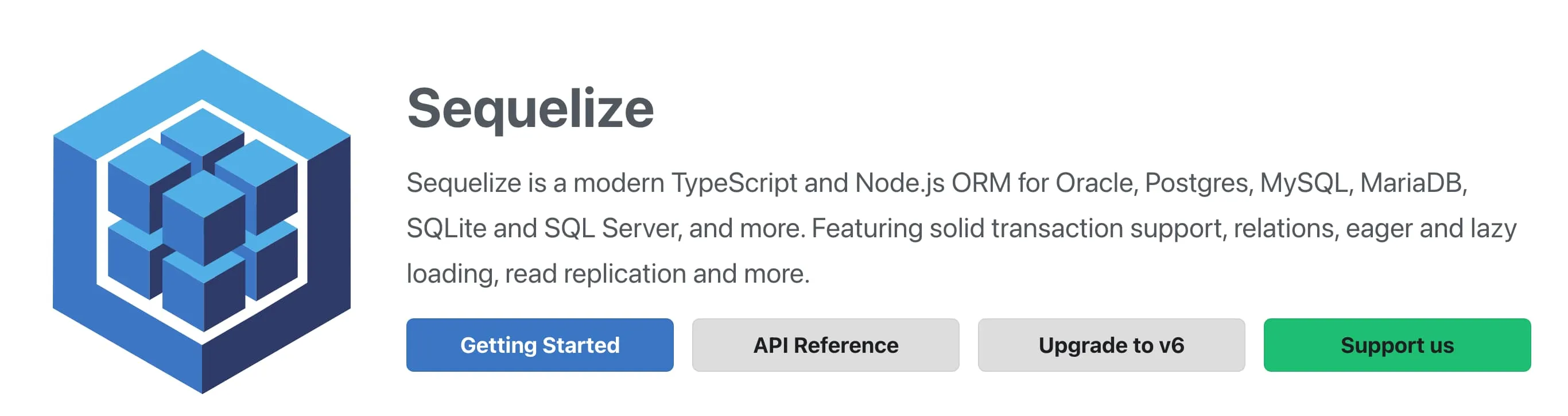
Key Features
- Supports various databases, including PostgreSQL, MySQL, SQLite, and MSSQL.
- Promise-based API.
- Migrations for database schema management.
Code Example
const { Sequelize, DataTypes } = require("sequelize");const sequelize = new Sequelize("sqlite::memory:");const User = sequelize.define("User", { name: { type: DataTypes.STRING, allowNull: false, },});(async () => { await sequelize.sync(); const users = await User.findAll(); console.log(users);})();
Documentation
Comparison of Prisma, TypeORM, and Sequelize
Feature/Tool | Prisma | TypeORM | Sequelize |
---|---|---|---|
Modern | Yes | Yes | Yes |
Lightweight | Yes | Yes | Yes |
Database Support | Extensive | Extensive | Extensive |
Database Compatibility | Strong | Strong | Strong |
Community Support | Strong | Strong | Strong |
TypeScript Support | Built-in | Built-in | Limited |
Pattern Support | Supports both Active Record and Data Mapper patterns | Supports both Active Record and Data Mapper patterns | Supports both Active Record and Data Mapper patterns |
API Style | Promise-based | Promise-based | Promise-based |
Type Safety | Generates TypeScript types to enhance safety | Generates TypeScript types to enhance safety | Limited |
Syntax Style | Expressive | Expressive | Verbose |
Visualization Interface | Prisma Studio | None | None |
Transaction Support | Strong | Strong | Strong |
Community Activity | Large and active | Large and active | Large and active |
Learning Curve | Relatively high (for beginners) | Relatively high (for beginners) | Relatively low |
Use Case | Ideal for projects with diverse database needs | Ideal for projects with diverse database needs | Suitable for traditional database operations |
Project Maturity | Relatively new, limited resources | Mature | Mature |
Conclusion
Choosing the right ORM for your project can be challenging. Here’s a quick guide based on different scenarios:
- Prisma or TypeORM: If your project has a long lifecycle, especially one involving TypeScript, Prisma should be your top choice. However, if you are already familiar with TypeORM or prefer a more flexible ORM, TypeORM is an excellent alternative.
- Sequelize: If you are working on a project that already uses Sequelize or requires specific features or libraries tied to Sequelize, consider sticking with it. Otherwise, TypeORM or Prisma might be more suitable.
While this article focuses on Prisma, TypeORM, and Sequelize, it’s worth noting that other options like MicroORM and Objection.js are available. However, their popularity and community support are not as extensive as the three discussed here. Additionally, if you’re using a non-relational database like MongoDB, an Object Document Mapper (ODM) like Mongoose would be a more appropriate choice.