Node.js, a highly regarded backend JavaScript runtime, has become the top choice for web developers worldwide. Not only is it one of the most popular programming languages, but it also significantly boosts development efficiency through code reuse via JavaScript libraries. However, with so many options available, selecting the right library for your project can be challenging.
Efficient libraries can dramatically speed up development and provide web applications with advantages like faster page loading and reduced application size. When choosing a library, developers should consider factors such as the application's complexity, community support, update frequency, and documentation quality.
Node.js libraries are managed via npm (Node Package Manager), which simplifies the installation of open-source libraries. Below, we introduce 13 hand-picked Node.js libraries, each with unique functionalities to streamline your web development process.
Overview of Node.js Libraries
A library, or module, is pre-written code that encapsulates commonly used functions. Libraries aim to accelerate coding, promote code reuse, and help developers adhere to the "DRY" (Don’t Repeat Yourself) principle. Unlike frameworks, which provide a structured program architecture, libraries offer specific functionalities that can be flexibly integrated at any stage of development.
13 Essential Node.js Libraries
Sequelize
Sequelize is a Promise-based ORM (Object-Relational Mapping) tool that simplifies interactions with relational databases like PostgreSQL, MySQL, MariaDB, and SQLite. It maps database tables to JavaScript objects, enabling developers to perform database operations without writing raw SQL queries. This reduces the risk of SQL injection and integrates seamlessly with GraphQL.
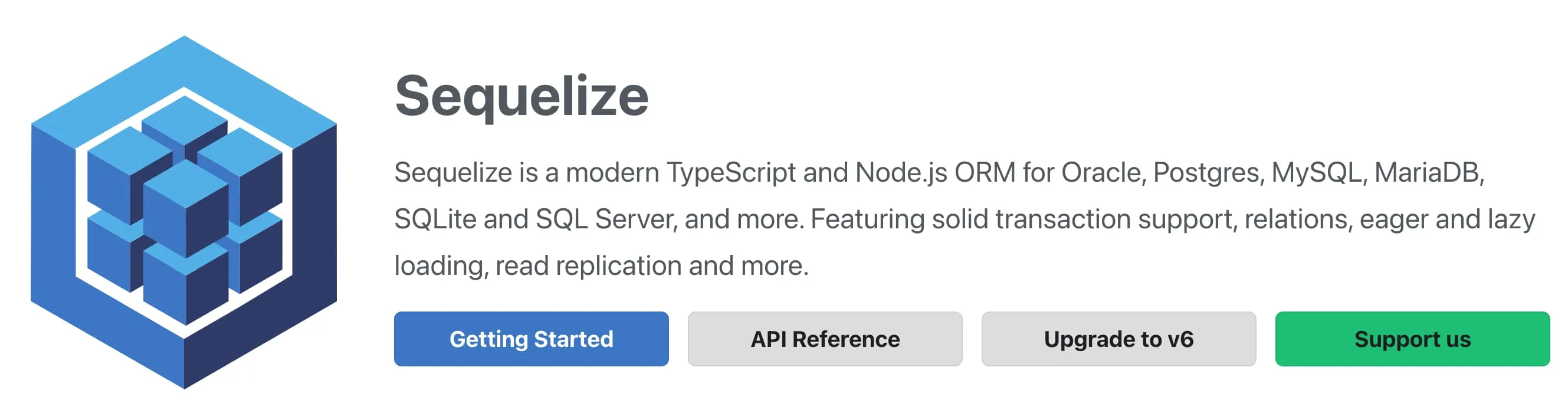
CORS
CORS (Cross-Origin Resource Sharing) is a middleware for Connect/Express that simplifies enabling cross-origin requests. It allows developers to specify allowed domains and provides flexible error-handling mechanisms to mitigate security risks.
var express = require("express");var cors = require("cors");var app = express();var corsOptions = { origin: "http://example.com", optionsSuccessStatus: 200,};app.get("/products/:id", cors(corsOptions), function (req, res, next) { res.json({ msg: "This is CORS-enabled for only example.com." });});app.listen(80, function () { console.log("CORS-enabled web server listening on port 80");});
Nodemailer
Nodemailer simplifies email sending in Node.js using the SMTP protocol. It supports multiple email services and allows developers to construct emails with parameters like from
, to
, and subject
, including HTML content.
Passport
Passport is an authentication middleware supporting over 500 strategies, including social media logins, OAuth, and OpenID. It streamlines the authentication process for web applications.
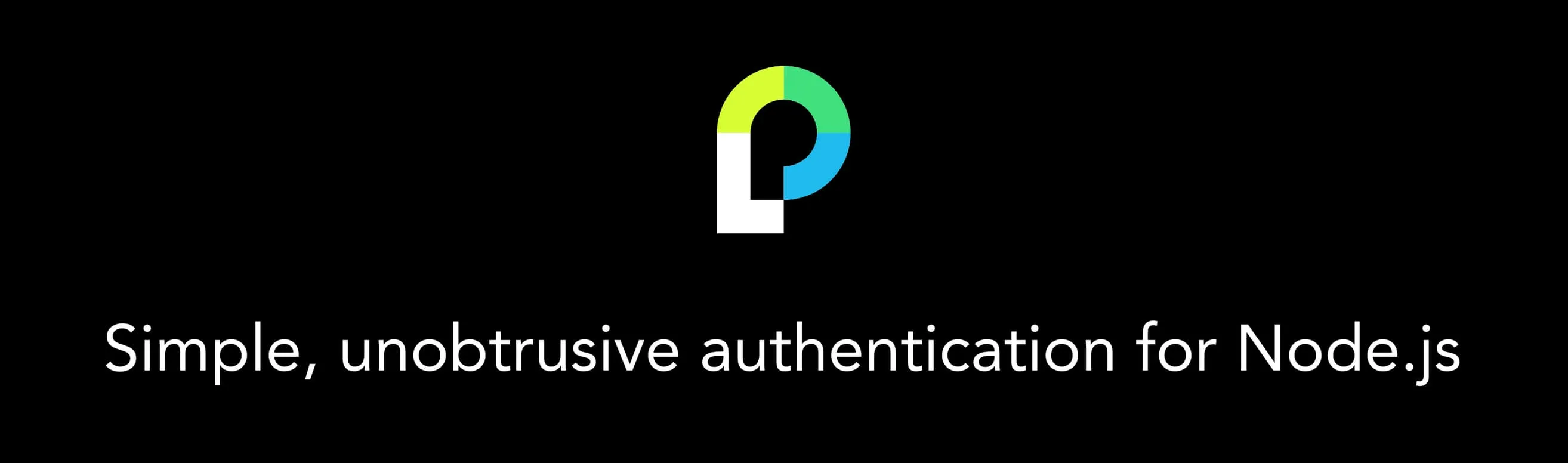
Async
Async is a utility module that simplifies handling asynchronous JavaScript. With over 70 methods, it helps developers avoid "callback hell" and manage asynchronous workflows efficiently.

Winston
Winston is a versatile logging library supporting multiple transport methods. It allows custom log formats and provides flexible log-level control.
const { createLogger, format, transports } = require("winston");const logger = createLogger({ level: "info", format: format.combine( format.timestamp({ format: "YYYY-MM-DD HH:mm:ss" }), format.errors({ stack: true }), format.splat(), format.json() ), defaultMeta: { service: "your-service-name" }, transports: [ new transports.File({ filename: "quick-start-error.log", level: "error" }), new transports.File({ filename: "quick-start-combined.log" }), ],});
Mongoose
Mongoose is an ODM (Object Data Modeling) library for MongoDB. It provides schema definition, model validation, and query building, offering a structured interface for MongoDB collections.
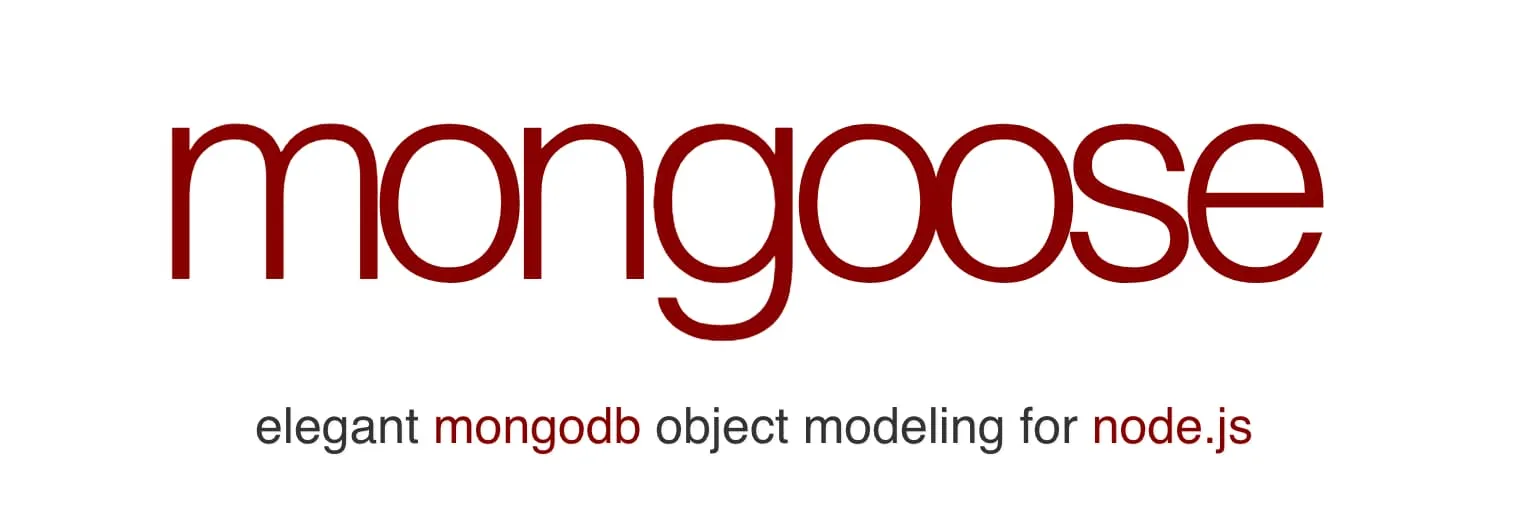
Socket.IO
Socket.IO enables real-time, bidirectional communication between servers and clients. It supports WebSocket and HTTP long-polling, making it ideal for scalable, event-driven applications.
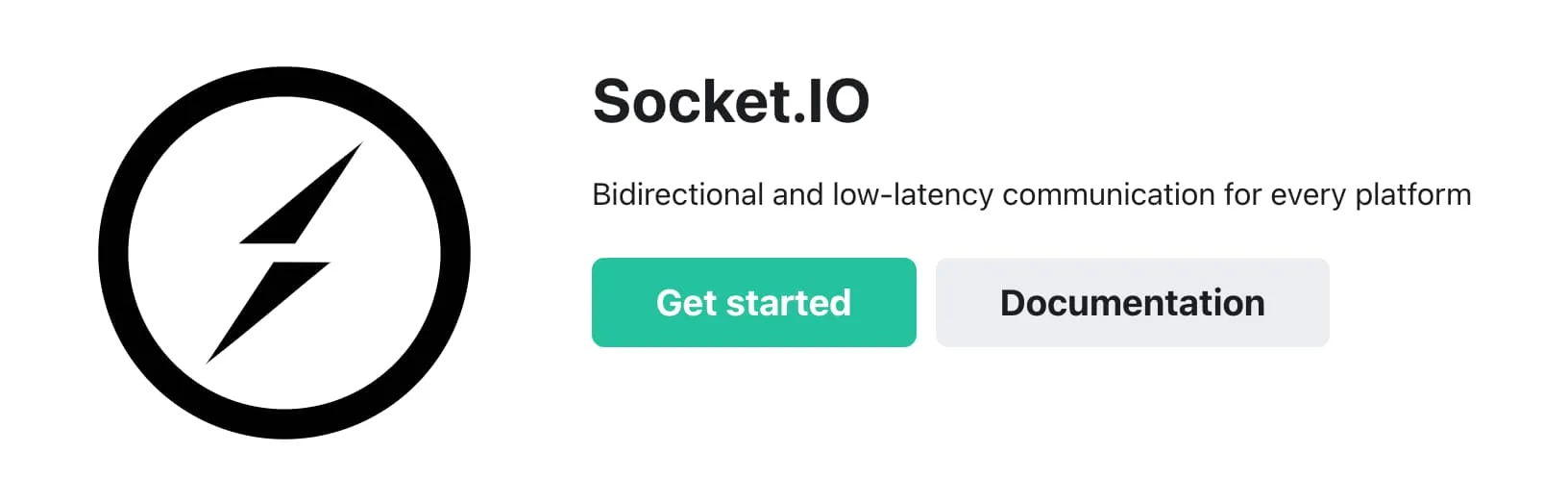
Lodash
Lodash is a utility library with over 200 functions for common programming tasks like type checking and mathematical operations.

Axios
Axios is a Promise-based HTTP client for Node.js and browsers. It supports automatic data transformation and includes security features to prevent CSRF attacks.
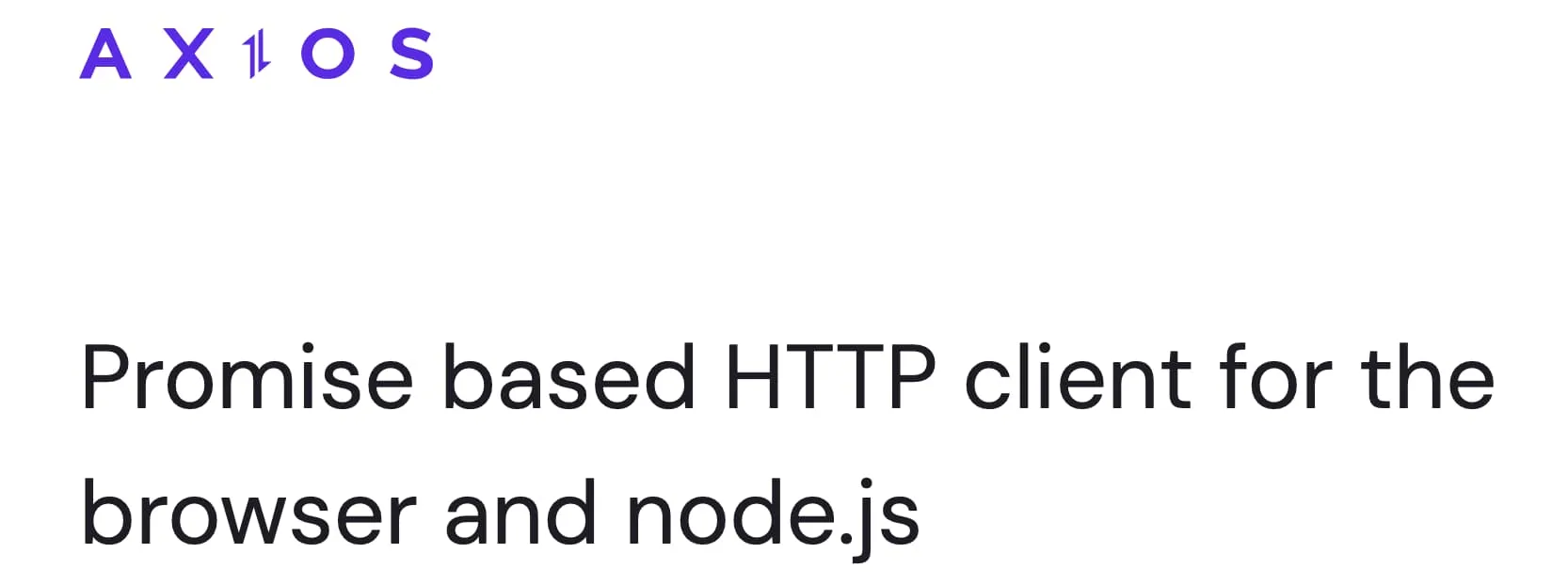
Puppeteer
Puppeteer is a Node.js framework for controlling Chrome/Chromium via the DevTools protocol. It’s widely used for automated testing and web scraping.
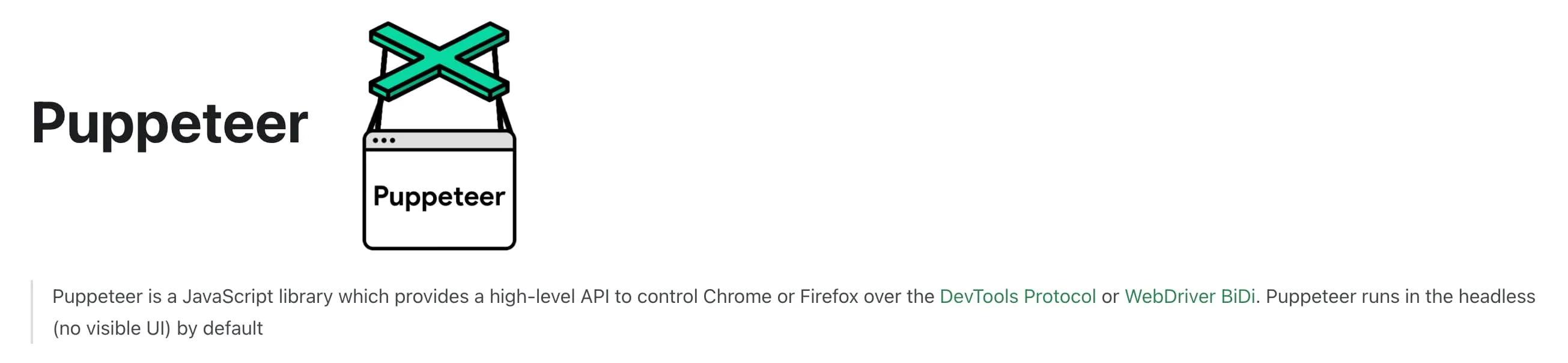
Multer
Multer is a middleware for handling multipart form data, particularly file uploads. Built on Busboy, it simplifies parsing and managing uploaded files.
const express = require("express");const multer = require("multer");const upload = multer({ dest: "uploads/" });const app = express();app.post("/profile", upload.single("avatar"), function (req, res, next) { // req.file is the 'avatar' file});
Dotenv
Dotenv manages environment variables, allowing developers to separate configuration data from source code. This enhances security and flexibility in application deployment.
Conclusion
The Node.js ecosystem offers a wealth of powerful libraries to streamline development. Choosing the right library is crucial for project success. The 13 libraries highlighted above can significantly enhance your next application, whether you're working with databases, authentication, or real-time communication. If you frequently use MongoDB, Mongoose is an excellent choice.